How To Generate A Service In Ionic
In Ionic Angular application contains the concept of ionic service: a class that contains business logic and information technology doesn't take UI. The service form contains methods and properties that can be reused past injecting them across application components, directives, and other services.
In this tutorial, we have two objective. Firstly how to manage | recollect| sending data to remote server using HTTP module. Second to allow sharing of data as simple demonstration of how nosotros tin can utilize service to shared data amongst related and non related component.
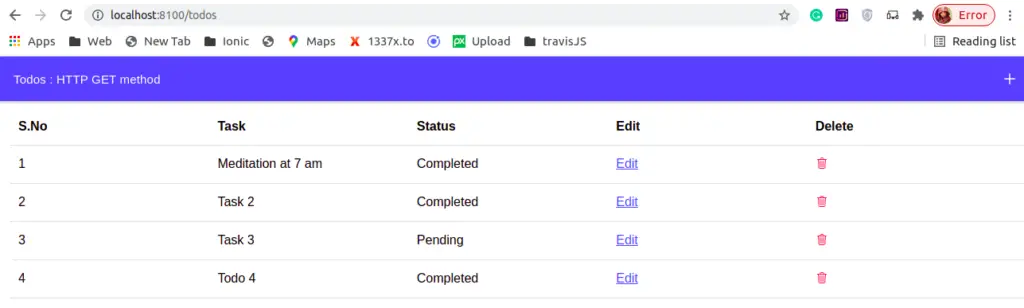
What is Service ?
Service is a course that acts equally a primal repository, as a central business unit nosotros could say something where you tin can store, where you can centralize code is shared across your application.
Angular services don't have a view and it holds shareable or reusable code in our applications. Here we have listed some of the most of import reasons why we need service in an Ionic application.
- Managing data land: For a small and medium application, we tin can use service and Ngrx library to manage the state of information across the awarding. In the large application, nosotros tin use Ngrx for reactive state management of information in Angular.
- Retrieving and sending data to remote server using REST API through Angular HTTP module.
- Centralize code containing business logic which shared accross application containing related and unrelated component.
- Sharing data: sharing data across components, especially among not related components (parent/child relationship amongst components
Setting and configure Ionic service instance. ?
We can create an ionic service manually or past using ionic CLI to generate a new service. Permit first create an Ionic application and use a bare template. Nosotros also need to create a service and we need a few pages to demonstrate HTTP requests.
ionic start sharedService blank --type=athwart cd serviceApp ionic generate service serviceName
Let create a todo, edit, and a new todo page, nosotros don't need a habitation folio. It is skillful practice to create a service binder for folio, service, model, and so on.
ionic one thousand page pages/todos ionic g folio pages/editTodo ionic g page pages/todo
In our HTTP asking, nosotros are performing a strict type request on different HTTP methods. For that, nosotros demand to create an interface of todo, let create binder models and add todo.model.ts file with the following type.
export interface ITodo { id?: string; task: cord; status: string; }
Configure routing module
We take created a few pages, we need to configure routing for these pages to demonstrate HTTP methods and permit edit the app.routing.module.ts file
import { NgModule } from '@angular/core'; import { PreloadAllModules, RouterModule, Routes } from '@angular/router'; const routes: Routes = [ { path: 'todos', loadChildren: () => import('./pages/todos/todos.module') .then(m => m.TodosPageModule) }, { path: '', redirectTo: 'todos', pathMatch: 'full' }, { path: 'todo', loadChildren: () => import('./pages/todo/todo.module') .then(chiliad => k.TodoPageModule) }, { path: 'todo/:id', loadChildren: () => import('./pages/edit-todo/edit-todo.module') .and so(k => chiliad.EditTodoPageModule) } ]; @NgModule({ imports: [ RouterModule.forRoot(routes, { preloadingStrategy: PreloadAllModules }) ], exports: [RouterModule] }) export class AppRoutingModule { }
Here is this ionic tutorial nosotros won't discuss on Ionic Angular routing, will only focus on service functionalities.
Import HTTP and Forms module
We need HttpClientModule and FormsModule to demonstrate how we tin use this module to demonstrate HTTP activities and permit edit the app.module.ts file.
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { RouteReuseStrategy } from '@athwart/router'; import { IonicModule, IonicRouteStrategy } from '@ionic/angular'; import { AppComponent } from './app.component'; import { AppRoutingModule } from './app-routing.module'; import { HttpClientModule } from '@athwart/mutual/http'; import { FormsModule } from '@angular/forms'; @NgModule({ declarations: [AppComponent], entryComponents: [], imports: [ BrowserModule, IonicModule.forRoot(), AppRoutingModule, HttpClientModule, FormsModule ], providers: [{ provide: RouteReuseStrategy, useClass: IonicRouteStrategy }], bootstrap: [AppComponent], }) export class AppModule {}
Create dummy information
We need a database where we can remember, update, delete and add together a new todo. Permit create dummy data in assets/information/todos.json
{ "todos": [ { "id": "1", "chore": "Meditation at seven am", "status": true }, { "id": "2", "task": "Task 2", "status": truthful }, { "id": "three", "task": "Task 3 ", "status": false }, { "id": "four", "chore": "Todo four", "condition": true } ] }
Setting remote server
Setting up the server and adding data to the database will make our tutorial very long. We'll useJSON Server topermit us to make fake HTTP requests without any coding and let install it globally.
In our Ionic service, we communicate remote server via HTTP client module, we can use the ngx-restangular library also. Nosotros tin apply an Angular FireModule, which is built specifically for Firebase. The AngularFire library uses Firebase's regular JavaScript/Web SDK under the hood
npm install json-server
All the HTTP requests to JSON Server are the same as Node-based express-js. To run the false server, nosotros need to run the command below on the terminal.
json-server src/assets/data/todos.json
Here is a screenshot of our fake server data.
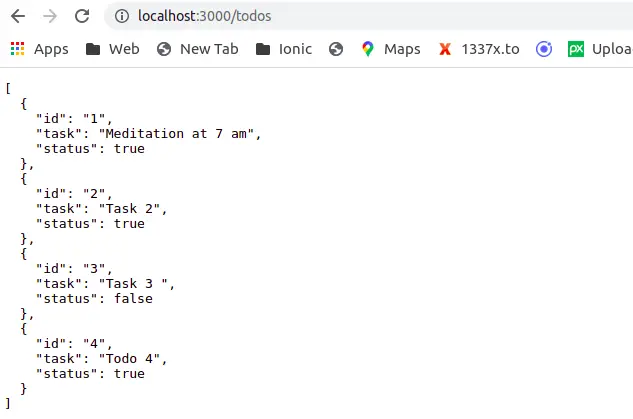
We accept already created a service class to request HTTP requests to a remote server. In the HTTP Service, we'll encapsulate some logic that helps manage the list of todos into service, edit, delete and create new todos using the HttpClient service from Angular.
import { Injectable } from '@athwart/core'; import { HttpClient, HttpErrorResponse } from '@angular/common/http'; import { Observable, throwError } from 'rxjs'; import { catchError } from 'rxjs/operators'; import { ITodo } from '../models/todo.model'; const URL_PREFIX = "http://localhost:3000"; @Injectable({ providedIn: 'root' }) export course TodosService { constructor(private http: HttpClient) { } getTodos(): Observable<Array<ITodo>> { return this.http.become<Array<ITodo>>(`${URL_PREFIX}/todos`,); } getTodo(id: cord): Observable<ITodo> { render this.http.go<ITodo>(`${URL_PREFIX}/todos/${id}`); } addTodo(todo: ITodo) { debugger render this.http.post(`${URL_PREFIX}/todos`, todo) .pipe( catchError((error: HttpErrorResponse) => { panel.log(fault.message); render throwError("Error while creating a todo" + fault.message); })); } updateTodo(todo: ITodo, id: string): Appreciable<ITodo> { render this.http.put<ITodo>(`${URL_PREFIX}/todos/${id}`, todo) .pipe( catchError((mistake: HttpErrorResponse) => { // this.errorHandler.log("Error while updating a todo", error); console.log(error.message); return throwError("Error while updating a todo " + error.bulletin); })); } deleteTodo(id: cord) { return this.http.delete(`${URL_PREFIX}/todos/${id}`).pipe( catchError((fault: HttpErrorResponse) => { console.log(error.message); render throwError("Mistake while deleting a todo " + error.message); })); } }
Consuming Ionic service for HTTP asking
We have learned that service classes contain reusable lawmaking and all our remote servers are kept in the service class and components are consumers of service. Now let first demonstrate how to apply HTTP Get method to call up all todo list in todos.component.ts file.
HTTP GET and DELETE method
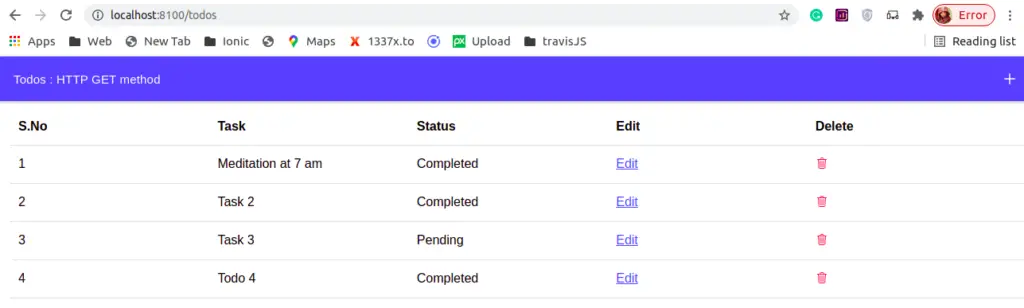
In the todo component template, we have used for structure directive to iterate through each loop. We too had two buttons, one to delete a todo and the edit push button will navigate us to the edit.todo.component to allow the edition of a todo.
import { Component, OnInit } from '@athwart/cadre'; import { ITodo } from 'src/app/models/todo.model'; import { TodosService } from 'src/app/services/todos.service'; @Component({ selector: 'app-todos', templateUrl: './todos.folio.html', styleUrls: ['./todos.page.scss'], }) consign class TodosPage implements OnInit { todos: ITodo[] = []; constructor(private todoService: TodosService) { } ngOnInit(): void { debugger this.todoService.getTodos() .subscribe((information: ITodo[]) => { this.todos = data }); } deleteTodo(todo: ITodo) { let id = todo.id ? todo.id : ''; this.todoService.deleteTodo(id).subscribe(() => { alert('Delete todo : '); }) } }
Edit the todos.component.html template to add ionic grid component to iterate through each todos listing.
<ion-header> <ion-toolbar color="primary"> <ion-title size="modest">Todos : HTTP Get method</ion-title> <ion-buttons slot="stop"> <ion-icon slot="start" name="add" routerLink="/todo"></ion-icon> </ion-buttons> </ion-toolbar> </ion-header> <ion-content> <ion-list> <ion-particular> <ion-grid fixed> <ion-row class="header"> <ion-col>S.No</ion-col> <ion-col>Job</ion-col> <ion-col>Condition</ion-col> <ion-col>Edit</ion-col> <ion-col>Delete</ion-col> </ion-row> </ion-grid> </ion-particular> <ion-item *ngFor="let todo of todos; index as i"> <ion-grid> <ion-row> <ion-col>{{ i + 1 }}</ion-col> <ion-col>{{ todo.job }}</ion-col> <ion-col> <span *ngIf="todo.condition">Completed</bridge> <span *ngIf="!todo.status">Pending</bridge> </ion-col> <ion-col> <a [routerLink]="'/todo/' + todo.id" size="small" shape="round"> Edit </a> </ion-col> <ion-col> <ion-icon (click)="deleteTodo(todo)" name="trash-outline" color="danger"></ion-icon> </ion-col> </ion-row> </ion-grid> </ion-item> </ion-list> </ion-content>
Http Postal service method
In our todo component, we take used the HTTP Mail method to add a new todo. Let edit the todo.component.ts file to implement to add a new todo.
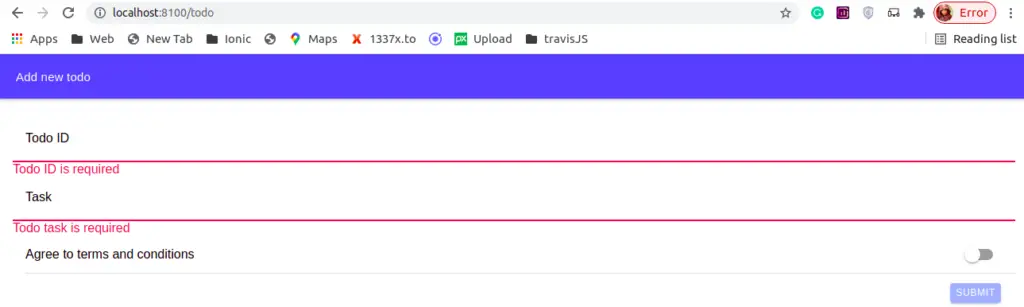
In a existent remote server, nosotros don't need to add together an id, this is fake server to demonstrate HTTP requests. Allow edit the todo component to implement adding new todo.
import { Component } from '@angular/core'; import { Router } from '@angular/router'; import { ITodo } from 'src/app/models/todo.model'; import { TodosService } from 'src/app/services/todos.service'; @Component({ selector: 'app-todo', templateUrl: './todo.page.html', styleUrls: ['./todo.page.scss'], }) export class TodoPage { todo: ITodo = { id: '', task: '', status: false } constructor( private router: Router, individual todoService: TodosService) { } addTodo(todo: ITodo) { this.todoService.addTodo(todo).subscribe(() => { alarm('Add new task successful' + todo); this.router.navigateByUrl('/'); }) } }
Let edit the todo component template to add a form to add a new todo to our remote server.
<ion-header> <ion-toolbar color="primary"> <ion-title size="pocket-size">Add new todo</ion-championship> </ion-toolbar> </ion-header> <ion-content> <div class="ion-padding"> <class #f="ngForm" novalidate (ngSubmit)="addTodo(f.value)"> <ion-listing> <ion-particular> <ion-label position="floating">Todo ID</ion-label> <ion-input type="text" proper name="id" required [(ngModel)]="todo.id" #idCtrl="ngModel" required></ion-input> </ion-item> <ion-text colour="danger" *ngIf="!idCtrl.valid && idCtrl.touched"> <ion-text colour="danger">Todo ID is required</ion-text> </ion-text> <ion-item> <ion-label position="floating">Task</ion-label> <ion-input type="text" name="task" required [(ngModel)]="todo.task" #taskCtrl="ngModel" required></ion-input> </ion-detail> <ion-text colour="danger" *ngIf="!taskCtrl.valid && taskCtrl.touched"> <ion-text color="danger">Todo task is required</ion-text> </ion-text> <ion-item> <ion-characterization>Agree to terms and conditions</ion-label> <ion-toggle [(ngModel)]="todo.status" name="condition" type="button"> </ion-toggle> </ion-item> <ion-item class="ion-float-right" lines="none"> <ion-button type="submit" size="pocket-sized" [disabled]="!f.valid"> Submit </ion-button> </ion-detail> </ion-list> </class> </div> </ion-content>
HTTP POST method
We can utilise the HTTP Post method to edit a todo, let edit the edit-todo.component.ts to implement HTTP Mail service methods.
import { Component, OnInit } from '@athwart/cadre'; import { ActivatedRoute, Router } from '@angular/router'; import { ITodo } from 'src/app/models/todo.model'; import { TodosService } from 'src/app/services/todos.service'; @Component({ selector: 'app-edit-todo', templateUrl: './edit-todo.page.html', styleUrls: ['./edit-todo.page.scss'], }) consign course EditTodoPage implements OnInit { todo: ITodo = { id: '', task: '', condition: simulated }; constructor( private router: Router, individual activatedRoute: ActivatedRoute, private todoService: TodosService ) { } ngOnInit() { permit id = this.activatedRoute.snapshot.params['id']; if (id) { this.todoService.getTodo(id).subscribe((data: any) => { this.todo = { id: data.id, task: data.task, status: data.condition } }) } } updateTodo(todo: ITodo) { this.todoService.updateTodo(todo, this.todo.id) .subscribe(() => { alert('Successful update'); this.router.navigateByUrl('todos'); }) } }
<ion-header> <ion-toolbar color="primary"> <ion-title size="small">Edit todo : {{ todo?.task}}</ion-championship> </ion-toolbar> </ion-header> <ion-content> <div form="ion-padding"> <grade #f="ngForm" novalidate (ngSubmit)="updateTodo(f.value)"> <ion-list> <ion-item> <ion-characterization position="floating">Todo ID</ion-label> <ion-input type="text" name="id" required [(ngModel)]="todo.id" #idCtrl="ngModel" required> </ion-input> </ion-item> <ion-text colour="danger" *ngIf="!idCtrl.valid && idCtrl.touched"> <ion-text color="danger">Todo ID is required</ion-text> </ion-text> <ion-detail> <ion-label position="floating">Task</ion-characterization> <ion-input type="text" name="task" required [(ngModel)]="todo.task" #taskCtrl="ngModel" required> </ion-input> </ion-detail> <ion-text color="danger" *ngIf="!taskCtrl.valid && taskCtrl.touched"> <ion-text color="danger">Todo task is required</ion-text> </ion-text> <ion-item> <ion-characterization>Agree to terms and weather condition</ion-label> <ion-toggle [(ngModel)]="todo.condition" name="status" blazon="button"> </ion-toggle> </ion-item> <ion-item form="ion-float-right" lines="none"> <ion-button type="submit" size="pocket-sized" [disabled]="!f.valid"> Submit </ion-button> </ion-item> </ion-list> </form> </div> </ion-content>
Example on how to use service in ionic to share data ?
In Ionic Athwart, the components can communicate with each other in many ways for parent/child-related components we can use input and fifty-fifty binding for sharing information.
With service, we can easily share data amid related and nonrelated components. In this example, we are creating a service called userService and by using this service when we change the data on the home folio and change data can exist displayed on a unlike page called confirm page.
On the dwelling page, nosotros display user information from the service and add together a new user data name and age field to the service user.service.ts. Nosotros have a confirmed push and clicking on confirm on the home page will route the states to confirm page where we can data of the dwelling house page from userService.
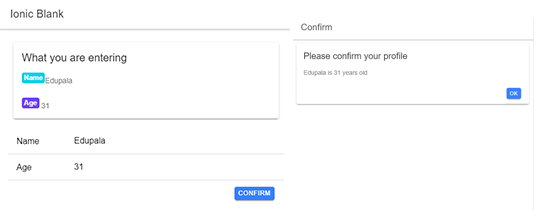
Create confirm folio and user service as
>> ionic generate page confirm
>> ionic generate service services/user
Edit user.service.ts file and add together go/set method to do additional processing when other pages admission or set the variables in this common class.
import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export grade UserService { individual _name: cord; individual _age: number; get name() { return this._name; } set name(proper noun: string) { this._name = name; } get historic period() { return this._age; } set age(age: number) { this._age = age; } }
Edit in home.page.html template file to add | edit user data.
<ion-header [translucent]="true"> <ion-toolbar> <ion-championship> Ionic vi Service </ion-championship> </ion-toolbar> </ion-header> <ion-content [fullscreen]="true"> <div class="ion-padding"> <ion-card> <ion-card-header> <ion-carte-championship>What yous are inbound</ion-card-title> </ion-bill of fare-header> <ion-card-content> <ion-badge colour="secondary">Proper name</ion-badge>{{ user.name }}<br /><br /> <ion-badge color="tertiary">Age</ion-badge> {{ user.age }} </ion-card-content> </ion-card> <ion-listing> <ion-item> <ion-label position="fixed">Proper noun</ion-label> <ion-input type="text" [(ngModel)]="user.name"></ion-input> </ion-item> <ion-particular> <ion-characterization position="stock-still">Age</ion-label> <ion-input type="number" [(ngModel)]="user.historic period"></ion-input> </ion-detail> <ion-item grade="ion-bladder-right" lines="none"> <ion-button color="chief" [routerLink]="'/confirm'">Confirm</ion-push> </ion-item> </ion-list> </div> </ion-content>
Edit n domicile.folio.ts file where nosotros have to inject userService service on our page so that we can use it.
import { Component, OnInit } from '@angular/cadre'; import { UserService } from '../user.service'; @Component({ selector: 'app-dwelling house', templateUrl: 'habitation.page.html', styleUrls: ['home.page.scss'], }) export class HomePage implements OnInit { user: any; constructor(private userService: UserService) { } ngOnInit() { this.user = this.userService; } }
Edit the confirm.page.html template to brandish data from the dwelling folio.
<ion-header> <ion-toolbar> <ion-title>Confirm</ion-title> </ion-toolbar> </ion-header> <ion-content> <ion-card> <ion-card-header> <ion-card-title>Please confirm your profile</ion-card-title> </ion-carte du jour-header> <ion-card-content> {{ user.name }} is {{ user.historic period }} years erstwhile </ion-card-content> <ion-item float-right> <ion-button colour="main" [routerLink]="'/home'">Ok</ion-push> </ion-item> </ion-carte du jour> </ion-content>
Edit ostend.page.ts file
import { Component, OnInit } from '@athwart/core'; import { UserService } from '../user.service'; @Component({ selector: 'app-confirm', templateUrl: './confirm.folio.html', styleUrls: ['./ostend.page.scss'], }) export grade ConfirmPage implements OnInit { user: any; constructor(private userService: UserService) { } ngOnInit() { this.user = this.userService; } }
Decision
We take completed the ionic service, why we demand it, and how we can use it to share information among dissimilar components. I promise yous got some idea on Ionic service usage and how to implement information technology.
Related posts
- How to implement an ionic slider
- Complete guide on an ionic grid with an example
- How to implement an ionic alert controller.
- How to implement an ionic table with pagination.
- How to used ionic skeleton component.
- How to create a dissimilar ionic listing.
How To Generate A Service In Ionic,
Source: https://edupala.com/ionic-service/
Posted by: arnoldexperwas89.blogspot.com
0 Response to "How To Generate A Service In Ionic"
Post a Comment